Apr 01 2021
Build Ansible Inventory from IBM Cloud Resources
IBM Cloud relies on Softlayer API – also called IBM Classic Infrastructure – to automate things. The API is available in different languages such as Python, Go, Java or PHP, and can be used to build an Ansible inventory.
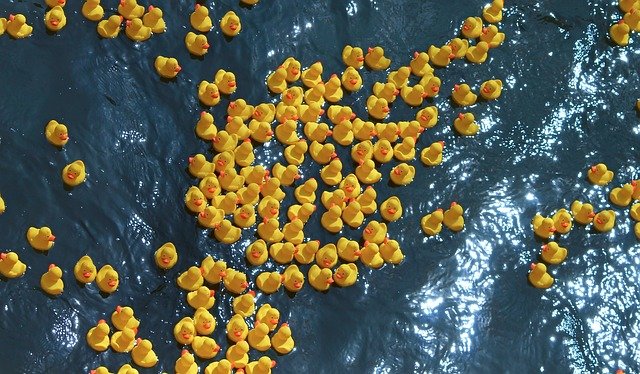
Here I will generate automatically a host inventory collected from my IBM Cloud account, directly usable by Ansible. I will also generate datacenters groups. You may want to fit servers in all kinds of categories such as Databases, Mysql, Linux, Web, etc… No worries, we can do that too in a really simple way.
This can be achieved adding tags to hosts on IBM Cloud portal and a host can indeed have multiple tags and belongs to Ansible groups matching these tags.
Once these groups are declared in the inventory file, you can apply Ansible playbooks like Install Apache package on servers that belong to the “Web” hostgroup for instance.
You first need to add your API key into a shell file you will execute before running the script. It could be in the script, but this allows each user to have his own key in a separate file. The API Key can be generated or found on IBM Cloud portal.
export SL_USERNAME=username
export SL_API_KEY=a1b2c3[...]3456
Run . ./IBM_SL_env.sh first to set username and API key into environment variables that will be used by the following Python script.
#!/usr/bin/env python3
import os
import SoftLayer
HOST_VARS_DIR = "host_vars"
INVENTORY_FILE = "inventory"
class Inventory:
def __init__(self):
# Variables
self.categories = {}
self.datacenter = {}
self.servers = {}
# Create Softlayer connection
self.client = SoftLayer.create_client_from_env()
# Init Methods
self.get_server_list()
self.write_inventory()
def short_host_name(self, host):
return host['fullyQualifiedDomainName'][:host['fullyQualifiedDomainName'].find('.mydomain.')]
def add_host_to_cat(self, host, cat):
if cat == "ibm-kubernetes-service": cat = "kube"
if cat not in self.categories:
self.categories[cat] = [host]
else:
self.categories[cat].append(host)
def add_server_to_list(self, host):
try:
host["primaryBackendIpAddress"]
except KeyError:
pass
else:
host["ShortHostname"] = self.short_host_name(host)
# Build server Categories list
if host["tagReferences"] != []:
for tagRef in host["tagReferences"]:
self.add_host_to_cat(host["ShortHostname"], tagRef["tag"]["name"].strip())
# Build datacenter lists
if host["datacenter"]["name"] not in self.datacenter:
self.datacenter[host["datacenter"]["name"]] = [host["ShortHostname"]]
else:
self.datacenter[host["datacenter"]["name"]].append(host["ShortHostname"])
# Build server attribute list
serverAttributes = {}
serverAttributes['IP'] = host["primaryBackendIpAddress"]
self.servers[host["ShortHostname"]] = serverAttributes
def get_server_list(self):
object_mask = "mask[id,fullyQualifiedDomainName," \
"primaryBackendIpAddress," \
"tagReferences[tag[name]]," \
"datacenter]"
# Get virtual server list
mgr = SoftLayer.VSManager(self.client)
for vs in mgr.list_instances(mask=object_mask):
self.add_server_to_list(vs)
# Get bare metal servers
hsl = SoftLayer.HardwareManager(self.client)
for hs in hsl.list_hardware(mask=object_mask):
self.add_server_to_list(hs)
def write_inventory(self):
# host_vars structure
if not os.path.exists(HOST_VARS_DIR):
os.makedirs(HOST_VARS_DIR)
inventoryFile = open(INVENTORY_FILE,"w")
for cat in self.categories.keys():
if cat != "kube":
inventoryFile.write("[" + cat + "]\n")
for host in self.categories[cat]:
# write host vars
inventoryFile.write(host + "\n")
file = open(HOST_VARS_DIR+"/"+host,"w")
file.write("ansible_host: " + self.servers[host]['IP'] + "\n")
file.close()
inventoryFile.write("\n")
for dc in self.datacenter.keys():
inventoryFile.write("[" + dc + "]\n")
for host in self.datacenter[dc]:
if not host.startswith("kube"):
inventoryFile.write(host + "\n")
inventoryFile.write("\n")
inventoryFile.close()
if __name__ == "__main__":
exit(Inventory())
A few notes:
Managed Kubernetes nodes are visible in IBM classic infrastructure like any other VM (VSI in IBM terminology) but you cannot connect on to them. They’re added to the “Kube” category before being ignored.
A distinct file is created in hosts_var for every server with its IP address. You could add more variables into it of course such as iSCSI settings, etc…
The script collects virtual machines as well as bare metal machines. You can comment out one of these sections if you don’t need it, saving one call to IBM.
Now you can build an Ansible inventory with IBM cloud hosts on the fly in less than 10 seconds.